Challenging Java Exercises

Java is a programming language used very widely in the real world. Here, you get to try taking the basic coding concepts you used in Scratch (operators, loops, and conditionals) to work through 7 activities involving "real code" programs! We will also explore the new, more advanced concepts of data types and functions.
Very unlike Scratch, in Java, you type and edit lines of code. And with the basics we show here, you can’t create art with sprites or backdrops. However, similar to Scratch, there’s still a window for editing the program. You also have a window where the computer displays the output of a program and talks with you, the programmer, or user.
In all of the activities below, don’t worry about all the words like "public," "class," "static," or "main." For now, just know they’re there for the computer to know where to begin running the program.
Some of these activities can get very challenging, especially near the bottom of the page. So take your time working through as many or as few of them as you'd like, at your own pace! But be sure to go in order from top to bottom. If you hit something confusing and get stuck, fear not, but if you want to know more, feel free to ask questions.
Note: To be able to see all the code, you may need to use the scroll bar on the side of the white code-editing window.
Writing a Short Story
In this activity, you will see how Java code "prints" (displays) words and sentences. A series of sentences has already been written for you. Following that format, in the code-editing window, try writing your own sentences in the space with the red line at the bottom. The words “System.out.print” will do the printing job for you, and “System.out.println” will print the sentence and start at a new line.
Click the green Run button at the top to run the program and test the code.
Printing with Loops
In this activity, you will work with while loops, often used Java, and one that you may have seen in Scratch. You will see them used to display the same sentence multiple times (repeating the sentence). Feel free to edit the raw code by changing how many times the sentence prints. You may also write your own sentence. Click the Run button to test the code.
True-or-False Math Equations
In this activity, the program is first given two numbers and a third number that we'll call a sum. The code tests whether or not those two numbers add together to equal the sum. In the code, you will see near the top a place where you can change the numbers and test it for yourself. Try to find out where the operators are. Click the Run button to test the code.
Quotients and Remainders
For this activity, first hit the green Run button. Then, without changing the code, type some numbers in the bottom display panel to test the function of the code. Directions will be printed out for you, and take a look at some of the operators shown in the code. The code will scan whatever numbers you type and return an answer in a new line. Click the Run button to test the code.
Prime Numbers
Prime numbers are numbers that only have two factors: 1 and the number itself. For example, 3 and 19 are prime numbers, but 15 and 4 are not. 15 has factors 3 and 5, and 4 has an extra factor of 2.
This activity allows you to test whether certain numbers are prime. You will put in a number of your choice, and the code will check it for you and return a statement. Look for conditional statements in the code! Remember not to put in numbers that are too big, or the code will crash! Click the Run button to test the code.
Rounding Pi (3.1415.....)
An Exploration with Functions
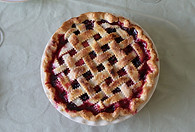

In Java, if we want to round something to the nearest whole number, we use this "operation" we call “Math dot round”.
Math.round(3.142) This example evaluates to 3.
But what if I wanted my program to round 3.142 to the tenths decimal place instead? Java doesn't give me a way to do that :(
The solution: Functions!
A function is a group of steps of code that does something specific. You can think of it as like an operation, but one that isn't already available. Instead, I have to create it myself, writing out the steps. Functions are also re-usable in your program.
You can create functions that do anything you want, and it doesn't have to be math! Maybe you'd like a function that makes the computer print out a greeting.
Below is a full function I've written, or defined, named “roundDecimal”. It uses variables and math operations. It lets us round any number out to a certain number of decimal places (decPlaces).
How does it work?
For example, here's how you can round 3.142 off to the hundredths place:
Step 1) multiply 3.142 by 100. The result is 314.2
Step 2) round that result off to the nearest whole number. The result is 314
Step 3) divide THAT result by 100. The final result is 3.14
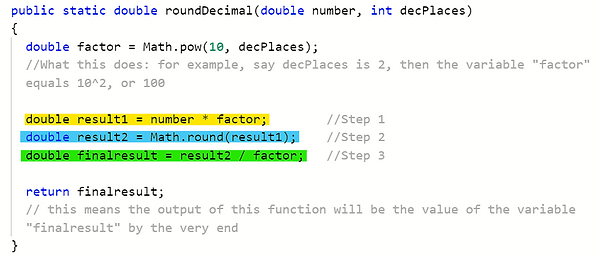
Once the function's steps are clear to you, here is something extra to think about:

This is what this Java code segment Math.pow(10, decPlaces) may look like in Scratch blocks, which together reads "10 to the power of decPlaces". Could we also create a variable named "factor" and give it this value in Scratch? Yes!
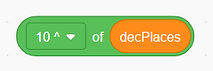
You can also make functions in Scratch. They are treated as custom-made blocks, found under the pink label "My Blocks". When starting a new pink block, you give it its own name and control what inputs it takes. See how the block I am about to make below looks very similar to the first line of Java code in the images above!
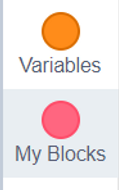
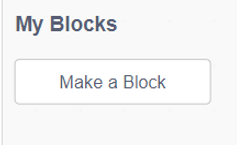
See our function in action within Scratch:
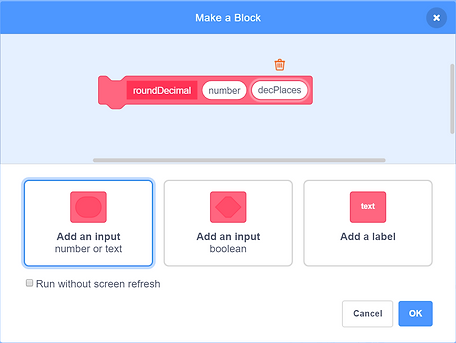
Scratch Connections?
The FINAL Challenge!
Circle Circumferences Using Different Pi's
This large project will tie every we've learned together. It will tell you how accurately you can find the circumference of a particular circle, as you try using different approximate values of Pi. Hit "run" to see it for yourself!
Try to figure out some of the code behind the scenes. You'll likely be able to spot where operations, loops, and conditionals are used. Can you find where our own roundDecimal function is used?
This is very complex and advanced! As you read the code, we do NOT expect you to understand every single line!
But to help you out a bit, here's a few more tips and a heads-up for other ideas that you can keep your eyes peeled for.
First of all, in previous Java activities above, have you been noticing the words "double," "int," "String," and "boolean" written in the code? These all have to do with variables, and hold important meaning. They're different data types -- read my notes within the code below to find out more.
Secondly, You might come across this phrase: Math.abs() It's is another math "operation" available in Java. It outputs the positive version of a number, also known as its distance from zero (its absolute value). You might notice in Scratch, that the block for this operation is named "abs" as well!